Here’s a baffling error that you might potentially run into when working with Audio Sources in Unity. Presenting the ArgumentNullException
:
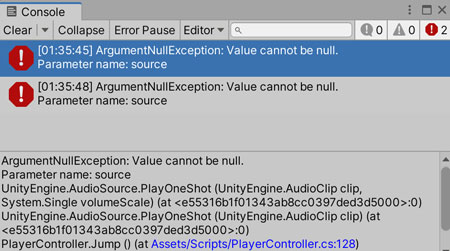
ArgumentNullException
on Audio Sources.Is your issue the same as mine?
If your error is like mine shown in the image above, with a part of the expanded error message saying that the error has someting to do with UnityEngine.AudioSource.PlayOneShot
, then this fix here should work for you. Otherwise, you may want to look somewhere else.
The solution
β¦is simple. You are simply missing an Audio Source component on the GameObject that also has your script causing the error. In my case, the error was caused by my PlayerController
script.
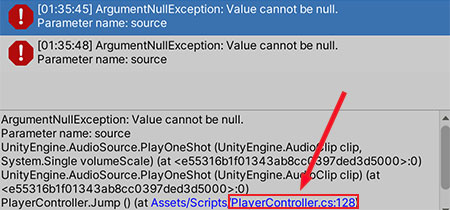
PlayerController
script was causing the error.So, just find the GameObject(s) in your scene with PlayerController
scripts attached to them, and make sure they have an Audio Source component too.
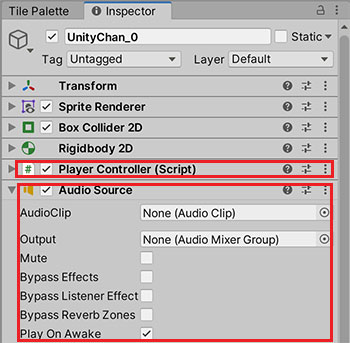
Why does this happen?
This happens because, in the example of my PlayerController
script, I use GetComponent()
to populate its audioSource
variable in Start()
. However, because I don’t have an AudioSource
component on the GameObject with my PlayerController
script, my audioSource
variable becomes null.
using UnityEngine; public class PlayerController : MonoBehaviour { AudioSource audioSource; void Start() { audioSource = GetComponent<AudioSource>(); } public void Jump() { audioSource.PlayOneShot(jumpSound); } void Update() { if(Input.GetButtonDown("Jump")) { Jump(); } } }
Hence, when I use the PlayOneShot()
method on my audioSource
, I am essentially doing null.PlayOneShot(mySound)
, which creates an error because you can’t do that with null objects.
If you are familiar with NullReferenceException
errors, this is essentially one. I am not sure why Unity documents them as an ArgumentNullException
error instead, because they are supposed to happen when you pass a null
as an argument to functions that do not accept them, such as in this example:
Instantiate(null);
It probably has to do with how the AudioSource
component’s functions work internally, but I will need to read to Unity’s source code to confirm that.
Conclusion
I hope this article helped you. If you have anything you’d like to add, please use the comments section below.