Recently, I came across an error that was quite the head-scratcher while grading some Unity scripts. Here’s what the error says:
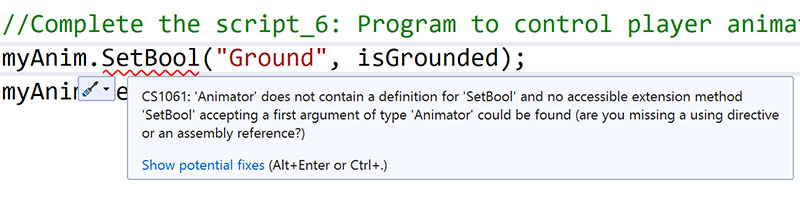
myAnim
variable was set with myAnim = GetComponent<Animator>()
earlier on. But SetBool()
is a valid method in the Animator
component from Unity, so what exactly is going on here?
Additionally, when I tried to use IntelliSense to find the method, this is what I got:
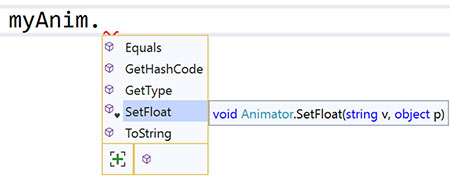
Animator
methods are missing.So most of the Animator
‘s methods and properties just seem to have decided to take a vacation, because the component usually has a much longer list:
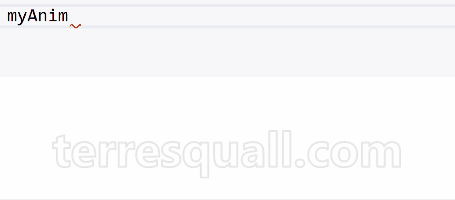
Animator
component comes with its substantial library of properties and methods.The issue: Another Animator
script in the project
It took me awhile to find the problem, but essentially, this issue was created because the Unity project had another Animator
class defined.
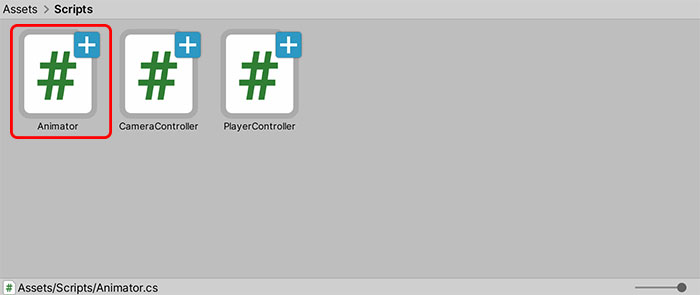
Animator
class defined in the projectThis alternate Animator
class had only a SetFloat()
method defined, and little else, as shown below. This is why I couldn’t access any of the usual Animator methods or properties.
Animator.cs
using System; internal class Animator { internal void SetFloat(string v, object p) { throw new NotImplementedException(); } }
To fix the error, all I had to do was delete the Animator
class defined in the project.
Why does this happen?
You’re probably wondering why this is possible. If we define an Animator
class in our own project, shouldn’t there be an error because this new, self-defined class is trying to take the same namespace as Unity’s Animator
class?
Well, this is possible because Unity’s Animator
class is actually under the UnityEngine
namespace, which means that the fully-qualified class name of the class is UnityEngine.Animator
. Hence, there are 2 ways to access the Unity Animator
:
using UnityEngine; public class ExampleClass { // The Animator here refers to UnityEngine.Animator, // because we are using UnityEngine. Animator anim; void Start() { anim = GetComponent<Animator>(); } }
// Without using the UnityEngine namespace, we will have // to use the fully-qualified class name. public class ExampleClass { UnityEngine.Animator anim; void Start() { anim = GetComponent<UnityEngine.Animator>(); } }
In my script where the error happened, because there are 2 Animator
classes defined, the self-defined Animator
and UnityEngine.Animator
, Unity always assumes that the self-defined Animator
is being used whenever the Animator
class is referred to, even when the script has the using UnityEngine
declaration on top.
Not the problem you are facing?
Now, if this article didn’t fix your problem, then your problem is likely much simpler than the one I faced. For starters, you should check if you’ve spelled the SetBool()
method correctly, and didn’t mis-spell it into something like Setbool()
or setBool()
.
Hope this article helped you!
Tkm <3