Are you looking to run Python as a server-side scripting language on your XAMPP installation? Here’s a guide on how to set it up.
1. Install Python
To begin, we have to make sure you have Python installed. Use one of the following commands in either your Command Prompt (Windows) or Terminal (macOS).
py --version
python --version
If the command outputs a version number, then Python is installed on your computer. Otherwise, you can download the Python installer here.
2. Add Python to XAMPP’s Apache
The next thing you need to do is find where Apache’s httpd.conf
is in XAMPP.
For Windows, you can open the XAMPP Control Panel and click on Config > Apache (httpd.conf).
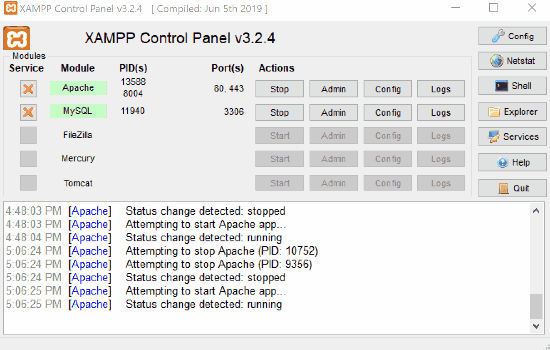
httpd.conf
in XAMPP.For macOS, you’ll need to click on the Open Application Folder button in the application. This brings you to the htdocs
folder within XAMPP. You’ll need to head one level up and find the apache/conf
folder — httpd.conf
will be in there.
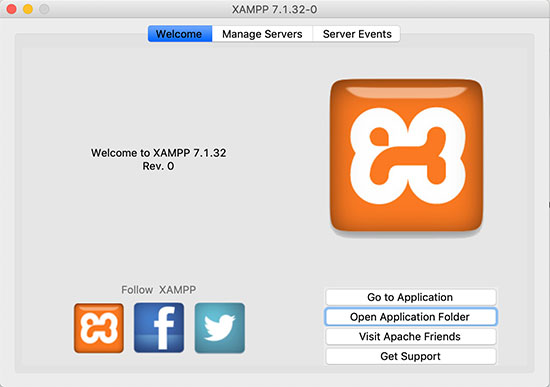
htdocs
folder. From there, you’ll need to head one level up, and head to apache/conf/httpd.conf
.3. Add support for .py
files
Once httpd.conf
is open (you can open it with any text editor, such as Notepad or TextEdit), add the following lines at the end of the file:
httpd.conf
AddHandler cgi-script .py ScriptInterpreterSource Registry-Strict
Optionally, if you want XAMPP to automatically load index.py
when a directory is accessed, find the following section in httpd.conf
and add the highlighted portion below:
httpd.conf
<IfModule dir_module> DirectoryIndex index.php index.pl index.cgi index.asp index.shtml index.html index.htm index.py \ default.php default.pl default.cgi default.asp default.shtml default.html default.htm default.py \ home.php home.pl home.cgi home.asp home.shtml home.html home.htm home.py </IfModule>
This will cause index.py
, default.py
or home.py
to be among the candidates to be loaded when a directory is accessed.
If you want Python files to only run on a specific site, you can also add the codes above into a <VirtualHost>
block, such as in httpd-vhosts.conf
.
4. Running your Python file
To test if your setup works, you can copy the following file into the htdocs
folder of your XAMPP installation. Note that you will have to replace the first line with the path to Python.
python.py (Windows)
#!C:\Program Files\Python39\python.exe
print("Content-Type: text/html\n\n")
print("Hello world! Python works!")
python.py (macOS)
#!/usr/bin/python
print("Content-Type: text/html\n\n")
print("Hello world! Python works!")
The first two lines need to be included for the Python file to work. The first line tells Apache which program to run to interpret the file, while the second line outputs the file as a webpage.
If you don’t know where Python is installed, you can run the following command on Terminal in macOS:
$ which python
For Windows, you’ll have to go to Start, search for Python, then Right-click > Open file location.
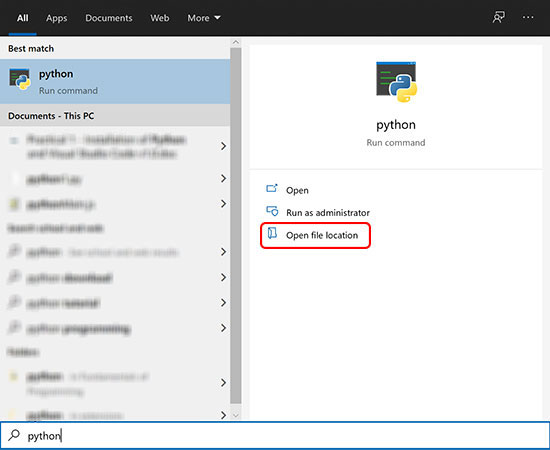
python.exe
in Windows.With the file in htdocs
, turn on Apache in XAMPP and you should be able to run the file by accessing http://localhost/python.py.
Make sure to restart Apache on XAMPP before doing any testing. This is so that the changes made to httpd.conf
will apply.
5. Importing Python PIP packages
If you’re looking to utilise additional packages from Python PIP, you’ll need to add some additional lines of code on your Python script(s).
import sys sys.path.append("[YOUR PACKAGES FOLDER]")
You’ll need to replace [YOUR PACKAGES FOLDER]
above with the place where your package(s) were installed. If you don’t know where this is, open Command Prompt or Terminal and type in the following:
pip show [YOUR PACKAGE NAME]
For example, to use the mysql-connector-python
package from PIP, pip show
gives the following output:
> pip show mysql-connector-python Name: mysql-connector-python Version: 8.0.27 Summary: MySQL driver written in Python Home-page: http://dev.mysql.com/doc/connector-python/en/index.html Author: Oracle and/or its affiliates Author-email: License: MySQL Connector/Python 8.0 license Location: c:\users\terresquall\appdata\roaming\python\python39\site-packages Requires: protobuf Required-by:
That means that I’ll have to add the following to the top of my Python script for my imported package to register:
python.py
#!C:\Program Files\Python39\python.exe
print("Content-Type: text/html\n\n")
import sys
sys.path.append("C:\\Users\\terresquall\\AppData\\Roaming\\Python\\Python39\\site-packages")
import mysql.connector
db = mysql.connector.connect(
host="localhost",
user="root",
password="",
database="testing",
auth_plugin='mysql_native_password'
)
cursor = db.cursor()
cursor.execute("SELECT * FROM `agents`;")
for row in cursor:
print("{0:10}\t{1:10}\t{2:10}\t{3:10}".format(row[0],row[1],row[2],row[3]))
6. Conclusion
If you couldn’t get Python to work, do share in the comments below the problem(s) you ran into. If we have a solution for you, we’ll respond to you in the comments!
If you’ve managed to solve your own problem, do share what you did to solve it too, so that other readers can make use of your solution.
I tried to import sys and got an error saying: Either the server is overloaded or there was an error in a CGI script
Hi Brilliant, you can check out this topic and see if it helps: https://stackoverflow.com/questions/33593410/either-the-server-is-overloaded-or-there-was-an-error-in-a-cgi-script
Thanks for this tutorial, it help me setup my python and its working well, although i did not import the PIP as you mention, i did under why i need to do that, although am just starting to learn python.
Good Day , I got the script to work on my windows system w.o. importing the sys module followed by the append path. The problem I have is that it won’t render in firefox but it will render in chrome. In firefox my py file just downloads. Can you think of a reason why this is? I followed all of your instructions. Thanks
Hi Josh, did you leave out the part that prints
Content-Type:text/html
? That tells it to render the file as a webpage. If you don’t include it, the browser is free to interpret your file however it wants, hence Firefox interpreting it as a download (Python files are not usually served as webpages).