If you’re making your foray into games programming, vectors are an important concept that you’ll have to understand and work with. If you’ve been reading build-your-own-game tutorials and getting confused by all the vector math that’s been going on, this article is probably a good one to read.
What are vectors?
Remember these diagrams you had to draw in secondary school?
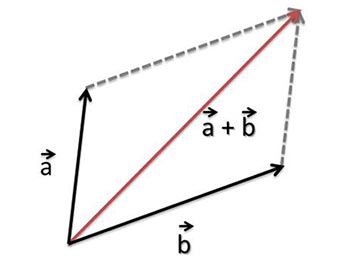
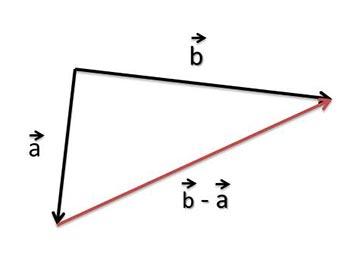
Well, these are vectors. They represent quantities in space. This means that they are like numbers, but they also have a direction. This makes them effective mathematical concepts for dealing with objects that are moving in 2-dimensional (2D) or 3-dimensional (3D) spaces.
The Cartesian coordinate system
In a programming context, vectors almost always exist inside a Cartesian coordinate system. This is a system of measuring space that uses 2 or 3 number lines to quantify 2D or 3D spaces, respectively.
A number line looks like this:
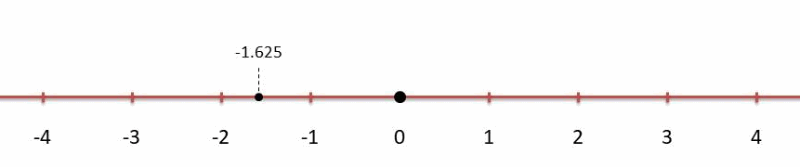
It is infinite in both directions, and every number is equally spaced from another. On a number line, you can identify a spot on the line by specifying a number.
While this may not be very useful, if you put 2 number lines together, you get a Cartesian coordinate system that can be used to represent 2D space. In such a system, we call the horizontal line the x-axis, and the vertical line the y-axis.
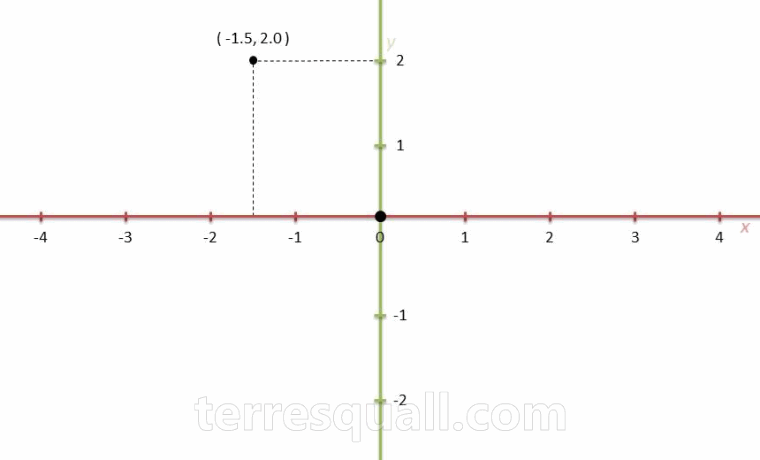
Adding a third number line (z-axis), as shown below, will give us a 3D coordinate system that can quantify 3D space.
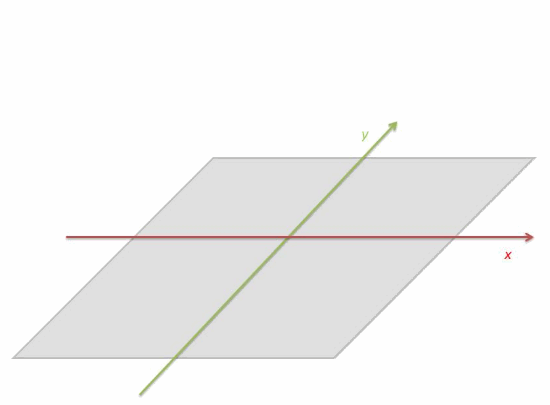
Article continues after the advertisement:
Vectors in coordinate systems
In a coordinate system, vectors are represented by a set of numbers. Vectors in a 2D coordinate system contain 2 numbers, while vectors in a 3D coordinate system contain 3 numbers. No surprises here. Each number in a vector represents the length of the vector along a given axis.
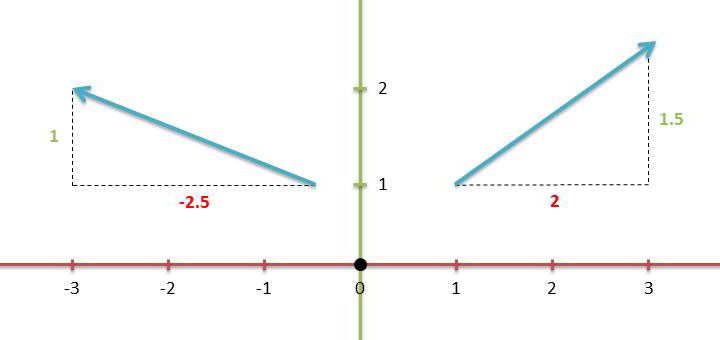
When dealing with vectors in Cartesian coordinates, you don’t need vector diagrams to add or subtract vectors. Simply sum up the numbers on each axis individually:
Vectors can also be multiplied or divided; but unlike addition and subtraction, they can only be multiplied by a single number, or a scalar.
Multiplying or dividing a vector changes its length without changing its direction. The vector gets longer or shorter, but still points where it used to point.
Vector lengths
To get the length of a vector, we can apply the Pythagoras Theorem. Notice that in a 2D vector, x and y form a right-angled triangle.
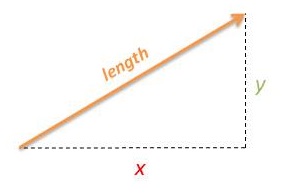
This means that you can get the vector’s length using the following formula:
Pythagoras Theorem works for 3D vectors too:
But there are handy shortcuts that you can use in modern game engines to find vector lengths, so you won’t have to apply the formula yourself. In Unity3D, you can simply use Vector2.magnitude
or Vector3.magnitude
properties. These will apply the Pythagoras theorem formula on your vectors to get you the length.
Vector2 v = new Vector(3,5); float dist = v.magnitude;
Vector3 v = new Vector(3,5,2); float dist = v.magnitude;
Article continues after the advertisement: