In Part 13, we added a damage feedback effect whenever the player receives damage.
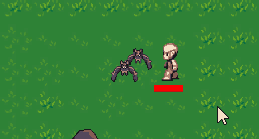
If you’d like to improve upon it by playing it on the location where the enemy touches the player, you’ll need to add an additional position
argument to the TakeDamage()
function on PlayerStats
:
public void TakeDamage(float dmg, Vector3? position = null)
{
//If the player is not currently invincible, reduce health and start invincibility
if (!isInvincible)
{
CurrentHealth -= dmg;
// If there is a damage effect assigned, play it.
if(damageEffect) Destroy(Instantiate(damageEffect, position ?? transform.position, Quaternion.identity), 5f);
invincibilityTimer = invincibilityDuration;
isInvincible = true;
if (CurrentHealth <= 0)
{
Kill();
}
UpdateHealthBar();
}
}
Essentially, what we're doing here is adding an optional argument to TakeDamage()
, so that if we provide a 2nd optional argument to it, it will play the effect on the position provided (instead of playing it on the player's position).
The position ?? transform.position
means we use position
if it exists, otherwise we use transform.position
.
Notice also that in the definition for the 2nd parameter, we have a ?
behind the Vector3
. This makes the Vector3
nullable, as they usually cannot contain a null value.
Once we modify the TakeDamage()
function, we now have to head to EnemyStats
and modify the OnCollisionStay2D()
function, where damage is dealt to the player:
void OnCollisionStay2D(Collision2D col)
{
//Reference the script from the collided collider and deal damage using TakeDamage()
if (col.gameObject.CompareTag("Player"))
{
PlayerStats player = col.gameObject.GetComponent();
player.TakeDamage(currentDamage, col.GetContact(0).point); // Make sure to use currentDamage instead of weaponData.Damage in case any damage multipliers in the future
}
}
Here, what we are doing is retrieving the first contact point between the enemy and the player, and providing the position of this point to our TakeDamage()
function. The contact point is retrieved from the col
parameter, which is provided to the function by Unity's own collision engine.
If you've implemented it right, the damage effect should now vary depending on where the enemy is touching you from.
View post on imgur.com