To complete our new weapon system for this series, we will need to have a proper buff / debuff system, because there are weapons in Vampire Survivors that apply buffs and debuffs to enemies. Hence, we're going to be working on this in Part 23, so we can create cool stuff like this:
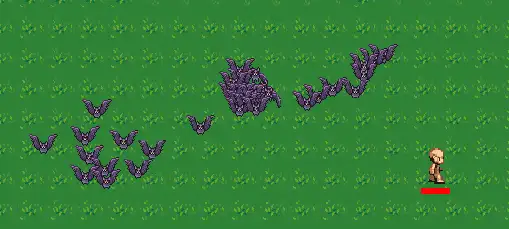
In 3F, is it meant to be “s1.damage += s2.damage” instead of adding maxHealth?