In Part 7 of our series, we created an enemy spawning system so that we had a way to generate enemies and run the core gameplay loop of our game. The enemy spawning system that we created then had a couple of limitations. For example, it was not possible to spawn the infamous Flower Wall that you saw in the Vampire Survivors game.
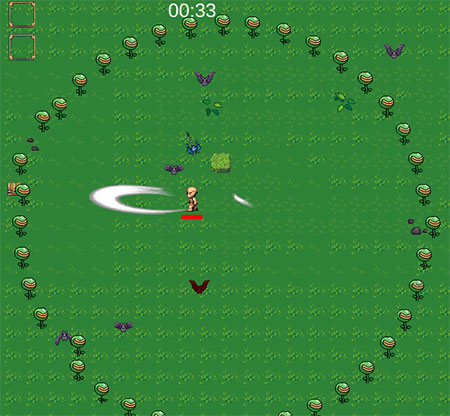
Hence, we're setting out to improve our enemy spawning system so that this sort of spawn becomes possible.
You may have missed the instruction for people to remove the three [HideInInspector] tags in 4A, or I missed the instruction to do so previously.